设计模式基础(12):组合模式&迭代器
包含组合模式&迭代器两种模式中的C++示例代码、面向的问题、图解两种模式核心思想
组合模式
面向的需求
- 对”部分-整体“这两种不同的对象,具有相同的使用接口。
示例代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80
| #include <iostream> #include <list> #include <string> #include <algorithm>
using namespace std;
class Component { public: virtual void process() = 0; virtual ~Component(){} };
class Composite : public Component{ string name; list<Component*> elements; public: Composite(const string & s) : name(s) {} void add(Component* element) { elements.push_back(element); } void remove(Component* element){ elements.remove(element); } void process(){ for (auto &e : elements) e->process(); } };
class Leaf : public Component{ string name; public: Leaf(string s) : name(s) {} void process(){ } };
void Invoke(Component & c){ c.process(); }
int main() {
Composite root("root"); Composite treeNode1("treeNode1"); Composite treeNode2("treeNode2"); Composite treeNode3("treeNode3"); Composite treeNode4("treeNode4"); Leaf leat1("left1"); Leaf leat2("left2"); root.add(&treeNode1); treeNode1.add(&treeNode2); treeNode2.add(&leaf1); root.add(&treeNode3); treeNode3.add(&treeNode4); treeNode4.add(&leaf2); Invoke(root); Invoke(leaf2); Invoke(treeNode3); }
|
代码思想分析
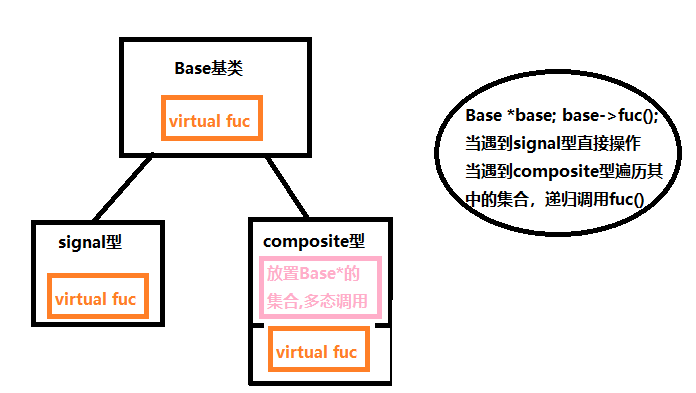
关键点
- 采用树形结构使得,之前对于多个类型的操作,统一为对一种类型的操作。
- 封装整体中的进一步细化操作,实现接口一致。
迭代器
面向的需求
- 面对不同的容器,不用关心其内部实现,为容器提供一套统一的调用接口。
示例代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| template<typename T> class Iterator { public: virtual void first() = 0; virtual void next() = 0; virtual bool isDone() const = 0; virtual T& current() = 0; };
template<typename T> class MyCollection{ public: Iterator<T> GetIterator(){ } };
template<typename T> class CollectionIterator : public Iterator<T>{ MyCollection<T> mc; public: CollectionIterator(const MyCollection<T> & c): mc(c){ } void first() override { } void next() override { } bool isDone() const override{ } T& current() override{ } };
void MyAlgorithm() { MyCollection<int> mc; Iterator<int> iter= mc.GetIterator(); for (iter.first(); !iter.isDone(); iter.next()){ cout << iter.current() << endl; } }
|
代码思想分析
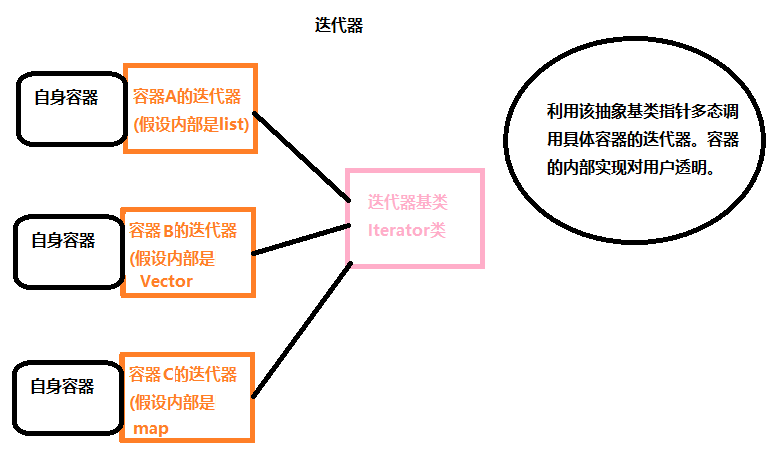
关键点
- 需要指出当前的设计思路为面向对象下迭代器设计,C++中目前多采用泛型编程的思想,这种方法已经逐渐抛弃。
- 但迭代器的总体思想还是不变的,为使用者提供一套统一接口,忽略容器内部实现的差异。